Delayed code execution with Unity's coroutines
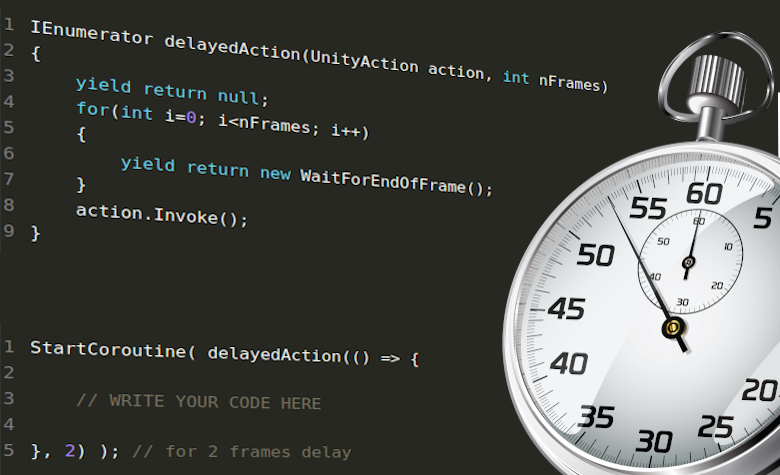
Sometimes a chunk of code must be executed one or more frames ahead, sometimes because it’s just convenient to do so, other times because your code simply wouldn’t work otherwise.
Mind that if you are in this situation, you should first ask yourself why. In fact, having to delay your code of one or more frames can be a symptom of engineering mistakes. But it may not depend on you. I had these situations in the past and the cause were most of the times external libraries or code from someone who left the company.
Unity exposes a very handy type called UnityAction to deal with delegates in a quick way. You almost don’t need any knowledge about delegates to use this approach, nonetheless I suggest you have a look at C# delegates.
A UnityAction may be roughly defined as a placeholder for a method. Below we define delayedAction Which accepts an UnityAction parameter. This allows us to pass our delegate which will be executed later in the body.
|
|
Now the way to use it might seem a bit strange if you never wrote an anonymous method. In C# anonymous methods are considered delegates and are compatible with UnityAction so we will use an anonymous method to pass any code to our delayedAction.
|
|
This would execute all code in the next frame.
Of course you can bend the delayedAction method to your needs. For example you might want to use WaitForSeconds, or you might want to wait for n frames before Unity executes the delayed code. In that case:
|
|
which would now be called as follows:
|
|